Working with SignalR and NCache Backplane
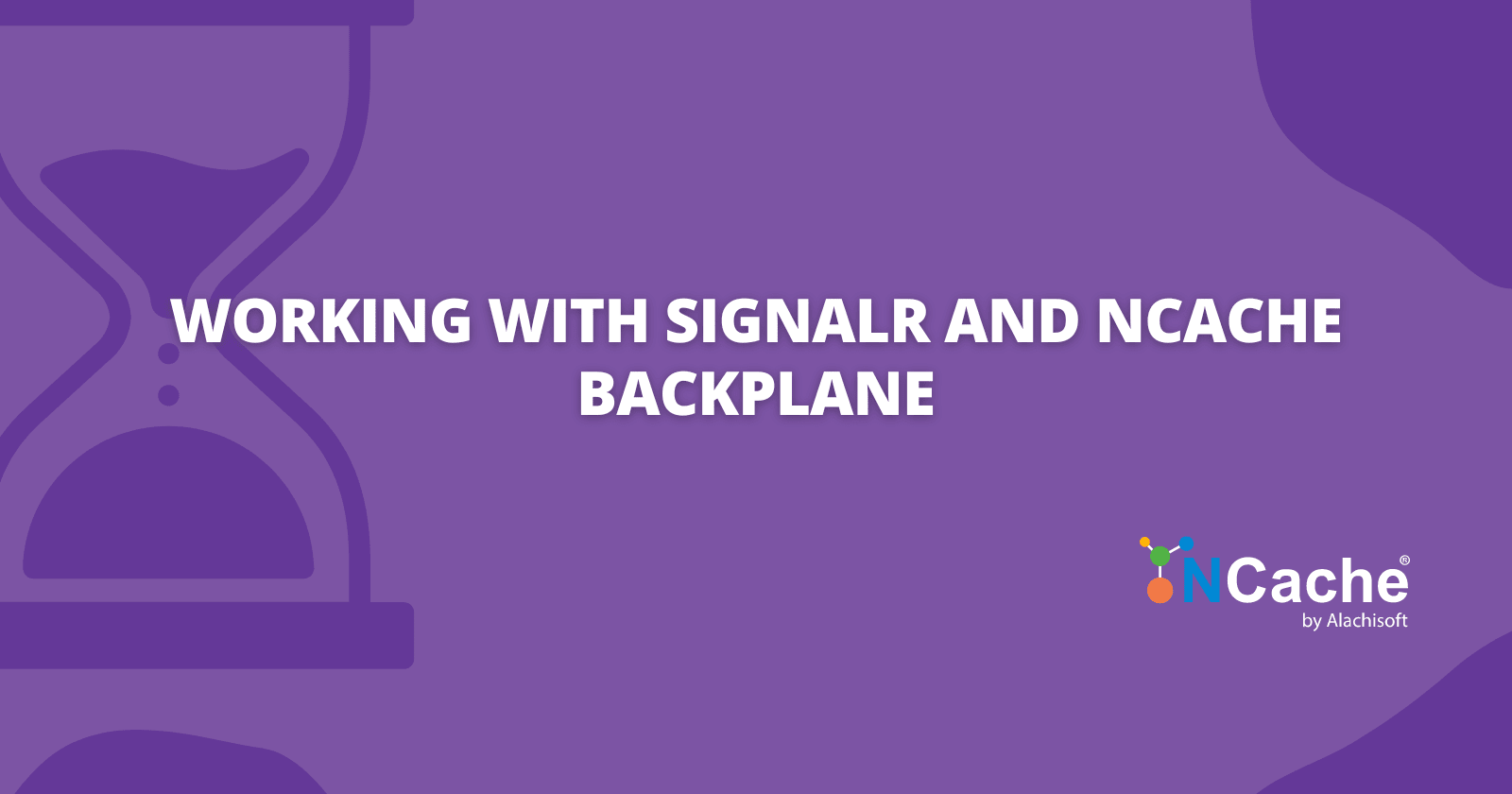
Real-time communication is a key requirement for modern web applications. Users expect fast and responsive applications that can provide real-time updates without the need to refresh the page. SignalR is a powerful technology that enables real-time communication between a client and a server, making it a popular choice for building real-time web applications.
However, as the user base grows, SignalR applications can become challenging to scale, especially when the number of clients and the amount of data being transmitted increases. This is where NCache comes in. NCache is a distributed in-memory caching solution that can be used to improve application performance and scalability.
In this article, we'll explore how to use SignalR and NCache together to build scalable and high-performance web applications. We'll start by introducing SignalR and NCache and their roles in web development. Then we'll show you how to use SignalR with NCache to improve web application performance. Finally, we'll discuss how to scale SignalR applications using NCache backplane.
Whether you're building a real-time chat application, a stock market ticker, or an online multiplayer game, the combination of SignalR and NCache can help you build high-performance, scalable, and real-time web applications. So, let's get started!
What is SignalR?
SignalR is an open-source library for ASP.NET that enables real-time communication between a client and a server. It allows developers to build real-time web applications that can push updates to clients as soon as the data changes on the server.
SignalR uses WebSockets to establish a persistent connection between the client and the server, enabling real-time communication. If WebSockets are not available, SignalR can automatically fall back to other transport mechanisms, such as Server-Sent Events (SSE), Long Polling, or Forever Frame.
SignalR provides a simple and easy-to-use API for building real-time web applications. It allows developers to send messages from the server to clients, from clients to the server, or even from client to client. This makes SignalR ideal for building applications that require real-time updates, such as online gaming, chat applications, or financial trading platforms.
SignalR also provides a robust error-handling mechanism that can handle connection failures, server timeouts, and other network issues. It also provides built-in support for scaling out, allowing you to scale SignalR applications across multiple servers.
Limitations of SignalR in a WebFarm
While SignalR is a powerful technology for building real-time applications, it can encounter certain limitations when implemented in a WebFarm environment. One of the main challenges of SignalR in a WebFarm is managing multiple servers and ensuring that messages are delivered correctly to all clients.
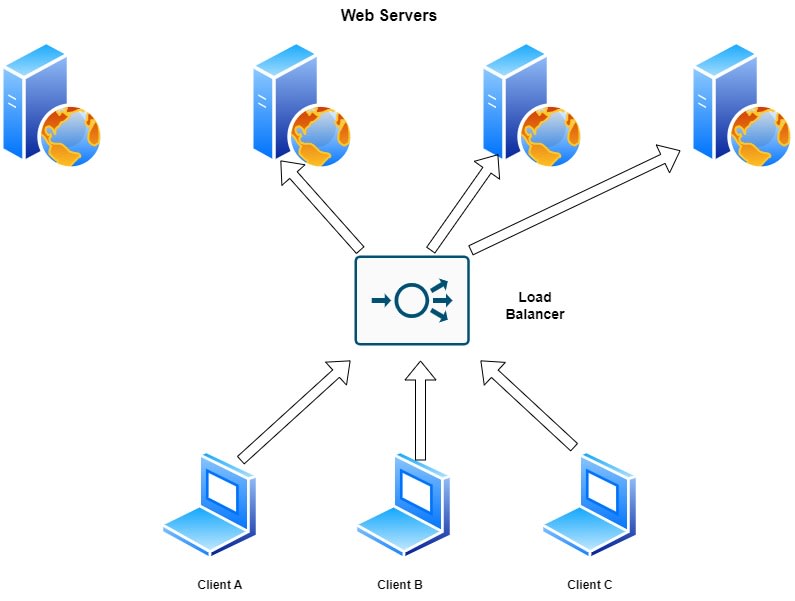
One major limitation is that SignalR relies on a single message bus for all servers in the WebFarm, known as the SignalR backplane. This can create a bottleneck when handling a large number of messages or clients and can result in delays or dropped messages. Additionally, SignalR's default load balancing algorithm is round-robin, which can lead to uneven distribution of messages among servers.
Another limitation of SignalR in a WebFarm is the inability to share in-memory data between servers. This means that if a client is connected to one server and then switches to another, it may not be able to retrieve the same data. This can lead to inconsistencies and incorrect data for the client.
To overcome these limitations, developers may need to implement workarounds or use third-party solutions such as NCache as a SignalR backplane. These solutions can help to distribute messages more evenly among servers and provide a shared cache for in-memory data. It's important to carefully consider the limitations of SignalR in a WebFarm and choose the appropriate solutions to ensure optimal performance and scalability for real-time applications.
Bottlenecks with SignalR Backplane
1. Real-time applications need less latency and more Throughput
- SignalR Backplane needs low latency and high Throughput.
- Database as SignalR Backplane is slow, Database can not scale out with increasing application load.
2. SignalR apps need guaranteed delivery of messages
- SignalR Backplane must be reliable.
- The database can choke down under extreme load and is a single-point failure.
3. SignalR Backplane must have high availability built into it
- SignalR backplane needs to be highly available.
- Backplane maintenance or unplanned outage can cause service delivery issues.
What is NCache?
NCache is an open-source distributed in-memory cache developed natively in .NET and .NET Core. NCache is a distributed cache that stores application data and prevents expensive database trips. It is extremely quick and linearly scalable. NCache can be used to reduce performance bottlenecks caused by data storage and databases, as well as to extend the capabilities of your .NET Core applications to handle high-volume transaction processing (XTP). It works both locally and configured as a distributed cache cluster for an ASP.NET Core app running in Azure or other hosting platforms.
NCache also provides built-in support for scaling out, allowing you to scale your application across multiple servers. It supports multiple cache topologies, including replicated, partitioned, and client-cache, enabling you to configure the cache to suit your application's needs.
Using SignalR with NCache
NCache stands out as an excellent option when considering third-party SignalR backplane providers due to several compelling reasons.
By combining SignalR with NCache, you can improve the performance and scalability of your real-time web applications. NCache can be used as a backplane for SignalR, enabling real-time updates to be shared across multiple servers.
When using NCache with SignalR, the client connections are distributed across multiple servers, and each server maintains its own connection to the NCache cluster. When a message is sent through SignalR, it is sent to the NCache cluster, which then distributes the message to all connected clients.
Using NCache as a backplane for SignalR offers several benefits.
- It improves the performance of real-time web applications by reducing the load on the database server.
- It enables high availability by distributing client connections across multiple servers, ensuring that clients can still receive updates even if one server fails.
- It enables scaling out by allowing you to add additional servers to the NCache cluster as your application grows.
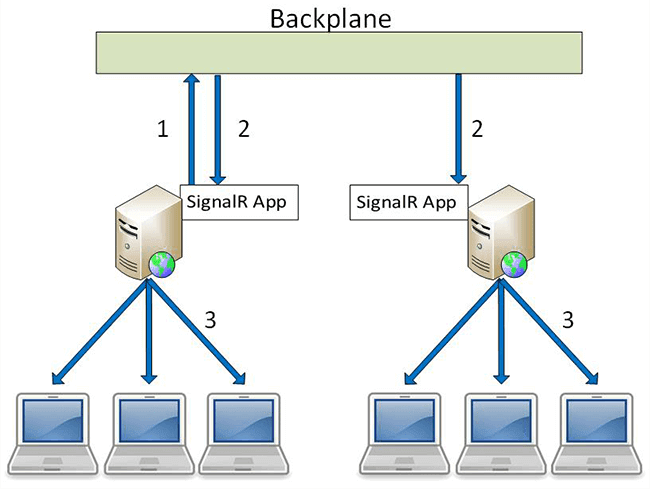
The main problem that NCache solves as a backplane is a scalability. NCache's distributed linear scalability enables each application to send messages to the backplane, and the backplane then sends the message back to the client's application. By eliminating the need to manage the complexity of parallel connectors, developers can focus on building robust applications without worrying about performance bottlenecks caused by backplane scalability limitations.
Scaling SignalR Apps with NCache Backplane
One of the key benefits of using NCache as a backplane for SignalR is the ability to scale out your application as your user base grows. NCache provides built-in support for scaling out, which allows you to add additional servers to the NCache cluster as your application grows.
When using NCache as a backplane for SignalR, client connections are distributed across multiple servers, ensuring that clients can still receive updates even if one server fails. This makes it possible to scale out your application by simply adding more servers to the NCache cluster. As new servers are added, the load is automatically distributed across all servers in the cluster, ensuring that each server can handle its fair share of the client connections.
Refer to the article Caching with NCache in ASP.NET Core for instructions on installing NCache and managing the cache using the NCache web monitoring application.
To begin the demo, we will use the existing ASP.NET Core SignalR application. The source code can be downloaded from GitHub.
The next step is to add a JSON object to the appsettings.json file.
"NCacheConfiguration": {
"CacheName": "mylocalcache",
"ApplicationID": "chatApplication"
},
CacheName: It is the name of the newly created cache
ApplicationID: should be a unique string relevant to the application.
To use NCache with SignalR, download and install the AspNetCore.SignalR.NCache
package from NuGet Package Manager. Then, add the below code to the Startup.cs
file. This code configures NCache options, including the cache name and application ID, using the values from the appsettings.json file.
services.AddSignalR().AddNCache(ncacheOptions => {
ncacheOptions.CacheName = Configuration["NCacheConfiguration:CacheName"];
ncacheOptions.ApplicationID = Configuration["NCacheConfiguration:ApplicationID"];
});
Start the application, then try to chat using two different user accounts. As soon as NCache starts, the NCache web monitor application will display the client count, as seen in the following figure:
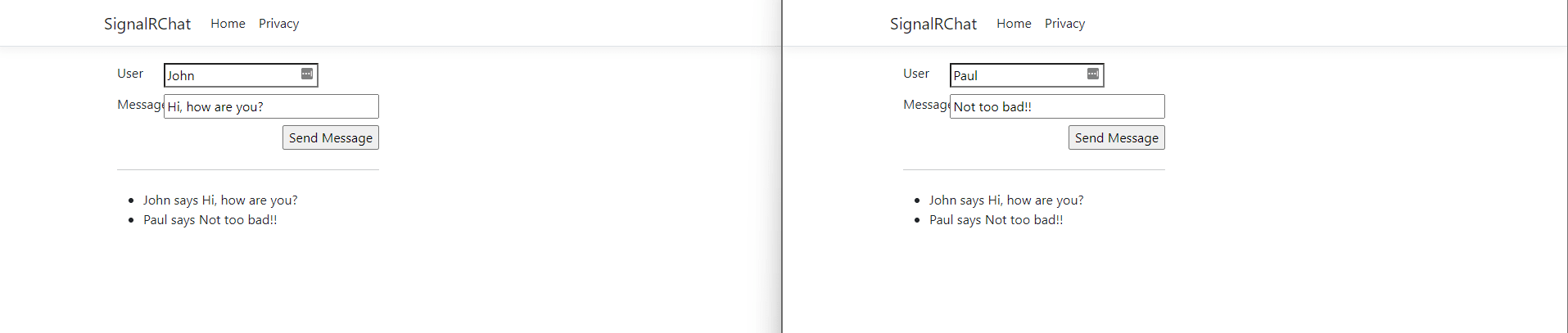
The client connection is now established, and our SignalR application is using the NCache as a backplane:
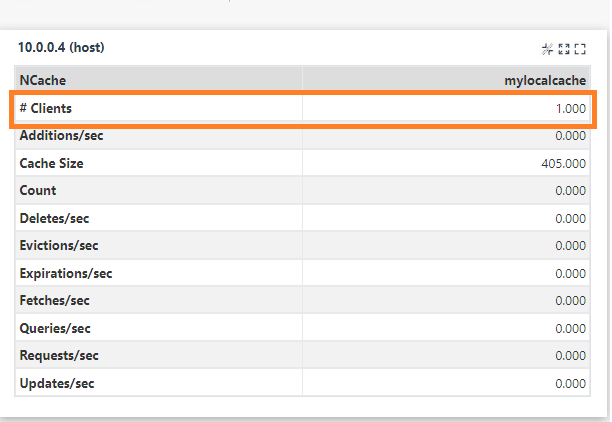
Conclusion
In conclusion, NCache with SignalR is a powerful combination for building real-time applications that are scalable, reliable, high-performing, and deliver real-time updates to clients. By leveraging the benefits of NCache and SignalR together, developers can build applications that provide a seamless user experience and can handle a high volume of data and traffic.
NCache provides several benefits, including scalability, high availability, performance, reliability, and reduced database load. Using SignalR with NCache enables real-time updates to clients, ensuring that they are always viewing the latest data. This makes NCache with SignalR a perfect fit for use cases such as financial applications, gaming applications, social media applications, and other real-time applications.